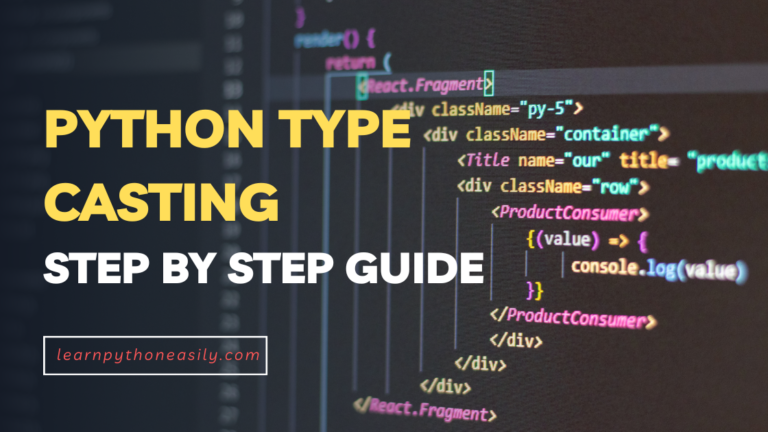
Type casting is the process of converting one data type to another data type. For example, you might have an integer value and you want to convert to a float or to a string or vice versa. Therefore Python provides several built-in functions or constructors that allow you to perform such conversions.
In this article, I will show you how to do Python Type Casting: Step By Step Guide.
Python Casting was performed using constructor functions including:
int()
: Converts a value to an integer.float()
: Converts a value to a float.str()
: Converts a value to a string.bool()
: Converts a value to a Boolean.
Table of Contents
Convert Float, String, and Boolean to Integer
To convert a float, string, or bool value to an integer we use a constructor function called int()
. This function takes an argument and returns an integer value.
Convert Float to Integer
In the following example, I will show you how to convert float to an integer using the int()
constructor.
Example:
# Convert float to an iteger
var_float = 4.6
# Use int() constructor function to convert float value to an integer
var_int = int(var_float)
# Print their value
print(var_float)
print(var_int)
print(int(7.90))
# Print the type
print(type(var_float))
print(type(var_int))
print(type(int(7.90)))
Output:
4.6
4
7
<class 'float'>
<class 'int'>
<class 'int'>
Convert String to Integer
In the following example, I will show you how to convert a string to an integer using the int()
constructor.
Note: In Python, type casting from a string to an integer only works if the string represents a valid integer value. But If the string contains non-numeric characters or has an invalid syntax, the type casting will not work and it will trigger a runtime error.
For example, we can convert the string "52"
to an integer using the int()
constructor function.
Example:
# Convert string to an iteger
# Use int() constructor function to convert string value to an integer
var_string = "52"
var_int = int(var_string)
# Print their value
print(var_string)
print(var_int)
print(int("7"))
# Print the type
print(type(var_string))
print(type(var_int))
print(type(int("7")))
Output:
52
52
7
<class 'str'>
<class 'int'>
<class 'int'>
The above example works successfully and converts the string literals to integers.
The following example will not work because we try to convert non-numeric characters to integers using the int()
constructor function, therefore such type casting will not work and it will trigger a runtime error.
Example:
# Convert string to an iteger
# Use int() constructor function to convert string value to an integer
var_string = "d52"
var_int = int(var_string)
# Print their value
print(var_string)
print(var_int)
print(int("*we3"))
# Print the type
print(type(var_string))
print(type(var_int))
print(type(int("*we3")))
Output:
Traceback (most recent call last):
File "c:\type_casting.py", line 38, in <module>
var_int = int(var_string)
^^^^^^^^^^^^^^^
ValueError: invalid literal for int() with base 10: 'd52'
The above code fails and it has resulted in an error saying “ValueError: invalid literal for int() with base 10: ‘d52‘”.
This error happens because we try to convert a string that contains non-numeric characters.
What about if the string contains a float number? Let us see this in an example.
Example:
# Convert string to an iteger
# Use int() constructor function to convert string value to an integer
var_string = "7.35"
var_int = int(var_string)
# Print their value
print(var_string)
print(var_int)
# Print the type
print(type(var_string))
print(type(var_int))
Output:
Traceback (most recent call last):
File "c:\type_casting.py", line 55, in <module>
var_int = int(var_string)
^^^^^^^^^^^^^^^
ValueError: invalid literal for int() with base 10: '7.35'
The above example does not work because the string "7.35"
contains a number with a decimal point. Therefore the compiler knows that what is inside the double quote is a float, not an integer. This is why we are getting the error saying “ValueError: invalid literal for int() with base 10: ‘7.35’“.
In the following example, I show you how to convert a string that contains a floating-point number to an integer, while using the correct procedure which is first to convert the string to float using thefloat()
constructor function, and then from float to integer, this truncates the decimal part after we use the int()
constructor function. Let us see this in an example.
Example:
# Convert string to an iteger
# Use int() constructor function to convert string value to an integer
var_string = "7.35"
var_float = float(var_string)
var_int = int(var_float)
# Print their value
print(var_string)
print(var_float)
print(var_int)
# Print the type
print(type(var_string))
print(type(var_float))
print(type(var_int))
Output:
7.35
7.35
7
<class 'str'>
<class 'float'>
<class 'int'>
Convert Boolean to Integer
In the following example, I will show you how to convert boolean (bool) type data to integer (int) using the int()
constructor function.
Note: The boolean values are only True
and False
. True is represented by 1 while False is represented by 0. Therefore if you convert True to int it will become 1 while the False becomes 0.
Example:
# Convert bool to an iteger
# Use int() constructor function to convert boolean values to an integer
var_bool = False
var_int = int(var_bool)
# Print their value
print(var_bool)
print(var_int)
print(True)
print(int(True))
# Print the type
print(type(var_bool))
print(type(var_int))
print(type(int(True)))
Output:
False
0
True
1
<class 'bool'>
<class 'int'>
<class 'int'>
Convert Integer, String, and Boolean to Float
To convert an integer, string, or bool value to a float we use a constructor function called float()
. This function takes an argument and returns a float value.
Convert Integer to Float
In the following example, I will show you how to convert an integer (int) to a float using the float()
constructor.
Example:
# Convert int to a float
# Use float() constructor function to convert integer values to a float
var_int = 45
var_float = float(var_int)
# Print their value
print(var_int)
print(var_float)
print(float(22))
# Print the type
print(type(var_int))
print(type(var_float))
print(type(float(22)))
Output:
45
45.0
22.0
<class 'int'>
<class 'float'>
<class 'float'>
Convert String to Float
In the following example, I will show you how to convert a string to a float using the float()
constructor function.
Note: In Python, type casting from a string to a float only works if the string represents a valid float value. But If the string contains non-numeric or non-float characters or has an invalid syntax, the type casting will not work and it will trigger a runtime error.
For example, we can convert the string "33.89"
to a float using the float()
constructor function.
Example:
# Convert string to a float
# Use float() constructor function to convert string values to a float
var_string = "33.89"
var_float = float(var_string)
# Print their value
print(var_string)
print(var_float)
print(float("22.56"))
# Print the type
print(type(var_string))
print(type(var_float))
print(type(float("22.56")))
Output:
33.89
33.89
22.56
<class 'str'>
<class 'float'>
<class 'float'>
Although the above code was successfully executed and converts the string literals to float. There are some cases where the code does trigger a runtime error.
The following example will not work because we try to convert non-numeric characters to float using the float()
constructor function, therefore such type casting will not work and it will trigger a runtime error.
Example:
# Convert string to a float
# Use float() constructor function to convert string values to a float
var_string = "ed33.89"
var_float = float(var_string)
# Print their value
print(var_string)
print(var_float)
print(float("e22.56"))
# Print the type
print(type(var_string))
print(type(var_float))
print(type(float("e22.56")))
Output:
Traceback (most recent call last):
File "c:\type_casting.py", line 123, in <module>
var_float = float(var_string)
^^^^^^^^^^^^^^^^^
ValueError: could not convert string to float: 'ed33.89'
The above code fails and it has resulted in an error saying “ValueError: could not convert string to float: ‘ed33.89’“.
This error happens because we try to convert a string that contains non-float or non-numeric characters.
What about if the string contains an integer number instead of a float number? Let us see this in an example.
Example:
# Convert string to a float
# Use float() constructor function to convert string values to a float
var_string = "8"
var_float = float(var_string)
# Print their value
print(var_string)
print(var_float)
print(float("44"))
# Print the type
print(type(var_string))
print(type(var_float))
print(type(float("44")))
Output:
8
8.0
44.0
<class 'str'>
<class 'float'>
<class 'float'>
Although the above code or string contains an integer value and we want to convert it to a float, thus, the code works and runs successfully. The float()
function just adds a decimal point to the number value (adds zero after the last digit in the number) found in the string and becomes a float.
Convert Boolean to Float
In the following example, I will show you how to convert boolean (bool) type data to a float using the float()
constructor function.
Note: The boolean values are only True
and False
. True is represented by 1 while False is represented by 0. Therefore if you convert True to a float it will become 1.0 while the False becomes 0.0.
Example:
# Convert bool to a float
# Use float() constructor function to convert boolean values to a float
var_bool = True
var_float = float(var_bool)
# Print their value
print(var_bool)
print(var_float)
print(float(False))
# Print the type
print(type(var_bool))
print(type(var_float))
print(type(float(False)))
Output:
True
1.0
0.0
<class 'bool'>
<class 'float'>
<class 'float'>
Convert Integer, Float, and Boolean to String
To convert an integer, float, or bool values to a string we use a constructor function called str()
. This function takes an argument and returns a string value.
Convert Integer to String
In the following example, I will show you how to convert an integer (int) to a string using the str()
constructor.
Example:
# Convert integer to a string
# Use str() constructor function to convert integer values to a string
var_int = 234
var_str = str(var_int)
# Print their value
print(var_int)
print(var_str)
print(str(783))
# Print the type
print(type(var_int))
print(type(var_str))
print(type(str(783)))
Output:
234
234
783
<class 'int'>
<class 'str'>
<class 'str'>
Convert Float to String
The following example shows how to convert a float to a string using the str()
constructor in Python.
Example:
# Convert float to a string
# Use str() constructor function to convert float values to a string
var_float = 234.587
var_str = str(var_float)
# Print their value
print(var_float)
print(var_str)
print(str(78.369))
# Print the type
print(type(var_float))
print(type(var_str))
print(type(str(783)))
Output:
234.587
234.587
78.369
<class 'float'>
<class 'str'>
<class 'str'>
Convert Boolean to String
The following example shows how to convert a boolean (bool) to a string using the str()
constructor in Python.
Example:
# Convert boolean to a string
# Use str() constructor function to convert bool values to a string
var_bool = True
var_str = str(var_bool)
# Print their value
print(var_bool)
print(var_str)
print(str(False))
# Print the type
print(type(var_bool))
print(type(var_str))
print(type(str(False)))
Output:
True
True
False
<class 'bool'>
<class 'str'>
<class 'str'>
Convert Integer, Float, and String to Boolean
In Python, a Boolean data type can only have two possible values, which are True or False.
To convert an integer, float, or string to a boolean (bool) value we use a constructor function called bool()
. This function takes an argument and returns a bool value which can be either True or False.
Convert Integer to Boolean
In the following example, I will show you how to convert an integer (int) to a boolean (bool) using the bool()
constructor.
Note: In Python, when converting an integer to a bool Python treats any non-zero integer to be evaluated as True, and zero will be evaluated as False.
Example:
# Convert integer to a boolean
# Use bool() constructor function to convert integer values to a boolean
var_int = 0
var_bool = bool(var_int)
# Print their value
print(var_int)
print(var_bool)
print(bool(676))
# Print the type
print(type(var_int))
print(type(var_bool))
print(type(bool(676)))
Output:
0
False
True
<class 'int'>
<class 'bool'>
<class 'bool'>
Convert Float to Boolean
In the following example, I will show you how to convert a float to a boolean (bool) using the bool()
constructor.
Note: In Python, when converting a float to a bool Python treats any non-zero float to be evaluated as True, and zero will be evaluated as False.
Example:
# Convert float to a boolean
# Use bool() constructor function to convert float values to a boolean
var_float = 0.0
var_bool = bool(var_float)
# Print their value
print(var_float)
print(var_bool)
print(bool(85.36))
# Print the type
print(type(var_float))
print(type(var_bool))
print(type(bool(85.36)))
Output:
0.0
False
True
<class 'float'>
<class 'bool'>
<class 'bool'>
Convert String to Boolean
In the following example, I will show you how to convert a string to a boolean (bool) using the bool()
constructor.
Note: In Python, when converting a float to a bool Python treats any non-empty string to be evaluated as True, and an empty string will be evaluated as False.
Example:
# Convert string to a boolean
# Use bool() constructor function to convert string values to a boolean
var_str = ""
var_bool = bool(var_str)
# Print their value
print("This is Empt", var_str)
print(var_bool)
print(bool("Hello"))
# Print the type
print(type(var_str))
print(type(var_bool))
print(type(bool("Hello")))
Output:
This is Empt
False
True
<class 'str'>
<class 'bool'>
<class 'bool'>
Conclusion
In this article, we have discussed how to do type casting in Python. We have also practically illustrated different kinds of performing type casting. This allows us to know how to convert one data type to another, such concepts and examples are important especially when we are working with multiple data types.
In detail, we have discussed four built-in constructors used when we perform type casting in Python such as int()
, float()
, str()
, and bool()
. We have learned how to use them with step by step practical guide. The articles provided a Python Type Casting: Step By Step Guide.
We highly encourage every Python beginners and intermediate programmers to learn and practice Python Type Casting during their Python learning journey.