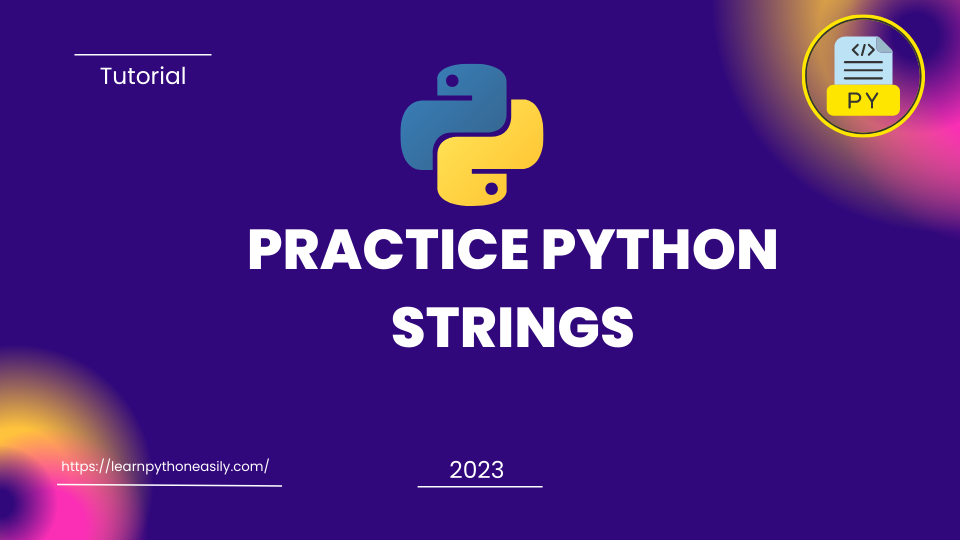
This tutorial is meant to provide a list of examples that will help you to practice Python Strings and fully understand some of the basics of Python Strings easily. The following subsections contain simple and easy-to-understand Python code dedicated to strings. Learn more about Python examples using this simple cheat sheet.
Table of Contents
Store String Values in a Variable
Example 1: Store string values in a Python variable.
str_one = "I love Python"
str_two = 'and I also like Django'
print(str_one, str_two)
Output:
I love Python and I also like Django
Store Singleline String Value in a Variable
Example 2: Similar to the above we store string values in a Python variable, this is also called single line string value and we cannot insert new lines.
str = "I love Python Because it easy to learn and easy to understand. Many of my friends like also Python language"
print(str)
Output:
I love Python Because it easy to learn and easy to understand. Many of my friends like also Python language
Try to insert a new line in the above string value and see what happens.
Example 3: Insert a new line in a single line string value.
str = "I love Python Because it easy to learn and easy to understand.
Many of my friends like also Python language"
print(str)
Output:
File "c:\Users\str.py", line 7
str = "I love Python Because it easy to learn and easy to understand.
^
SyntaxError: unterminated string literal (detected at line 7)
Python triggers an error if you try to insert a new line in a single line string value.
Store Multiline String Value in a Variable
Example 4: Store Multiline string values in a Python variable. Use triple quotes, single or double.
str_one = """I love Python Because it easy to learn and easy to understand.
Many of my friends like also Python language.
My skills are amazing."""
str_two = '''Similar to the above this is also a multiline string value
stored in Python variable.
Please practice this'''
print(str_one)
print(str_two)
Output:
I love Python Because it easy to learn and easy to understand.
Many of my friends like also Python language.
My skills are amazing.
Similar to the above this is also a multiline string value
stored in Python variable.
Please practice this
Check String Data Type
Example 5: Use the type()
function to check string type in Python.
str = "I love Python Because it easy to learn and easy to understand"
type_check = type(str)
print(type_check)
print(type("Python"))
print(type("10"))
Output:
<class 'str'>
<class 'str'>
<class 'str'>
Check String Length
Example 6: Use len()
function to check the total number of characters contains in a string.
str = "I love Python Because it easy to learn and easy to understand"
length_check = len(str)
print(length_check)
print(len("Python"))
print(len("10 0 [p ]"))
Output:
61
6
9
String Slicing
Example 7: Index and select the first, second, third, and last character of the string.
str = "Python is it easy to learn and easy to understand"
print(str[0])
print(str[1])
print(str[len(str)-1])
Output:
P
y
d
Example 8: Index and select the first half characters of the string.
str = "Python is it easy to learn and easy to understand"
print(str[:int(len(str)/2)])
Output:
Python is it easy to lea
Example 9: Index and select the last half characters of the string.
str = "Python is it easy to learn and easy to understand"
print(str[int(len(str)/2):])
Output:
rn and easy to understand
Example 10: Index and select the strings ranging from the first 20% to the last 70% of characters of the string.
str = "Python is it easy to learn and easy to understand"
print(str[int(len(str)*0.2):int(len(str)*0.7)])
Output:
it easy to learn and eas
Example 11: Slicing from the start (do not specify the start position/index, by default is 0) and slicing to the end (do not specify the last position/index, by default is the last index).
str = "Python is it easy to learn and easy to understand"
# Slicing from the start.
print(str[:20])
print(str[0:20])
# Slicing to the end.
print(str[20:])
print(str[20:len(str)])
Output:
Python is it easy to
Python is it easy to
learn and easy to understand
learn and easy to understand
Negative indexing: We use negative numbers to index, this indexing starts from the end of the sequence and counts backward.
Example 12: Using a negative number at the beginning of the slicing means slicing will start from the end of the string.
str = "Python is it easy to learn and easy to understand"
print("The last character of the string is :",str[-1:])
print("The last character of the string is :",str[-1:len(str)])
Output:
The last character of the string is : d
The last character of the string is : d
Example 13: Using negative numbers display the last three characters of the string using string slicing in Python.
str = "Python is it easy to learn and easy to understand"
print(str[-3:])
print(str[-3:len(str)])
Output:
and
and
Example 14: Using string slicing in Python display all the string characters except for the last character.
str = "Python is it easy to learn and easy to understand"
print(str[0:-1])
print(str[:len(str)-1])
Output:
Python is it easy to learn and easy to understan
Python is it easy to learn and easy to understan
Example 15: Display the last 50 percent of the string characters using negative indexing or slicing in Python.
str = "Python is it easy to learn and easy to understand"
print(str[-int(len(str) * 0.5):])
Output:
n and easy to understand
Combine String and Numbers
In Python, you cannot combine or concatenate string values into numbers (int and float) or boolean (bool) using the additional operator +
. If you do so, it will result in an error.
Example 16: Combining string and int using +
operator.
var_str = "Python is it easy to learn and easy to understand"
var_int = 90
print(var_str + var_int)
Output:
Traceback (most recent call last):
File "/Users/str.py", line 105, in <module>
print(var_str + var_int)
TypeError: can only concatenate str (not "int") to str
Example 17: Combining string and float using +
operator.
var_str = "Python is it easy to learn and easy to understand"
var_float = 89.67
print(var_str + var_float)
Output:
Traceback (most recent call last):
File "/Users/str.py", line 105, in <module>
print(var_str + var_int)
TypeError: can only concatenate str (not "float") to str
Example 18: Combining string and boolean (bool) using +
operator.
var_str = "Python is it easy to learn and easy to understand"
var_bool = True
print(var_str + var_bool)
Output:
Traceback (most recent call last):
File "/Users/str.py", line 105, in <module>
print(var_str + var_int)
TypeError: can only concatenate str (not "bool") to str
Example 16 to Example 18 has failed due to illegal concatenation. To correct the above code we can use a new Python feature called String Formatting by using f-Strings or format() method.
String Formatting
String formatting refers to the process of creating a string that contains all kinds of data values in a specified format. For example, you want to concatenate a sequence of string characters into numerical data without using Python’s string concatenation sign '+'
.
There are several options we can adopt to format our string and combine several data types in it using either f-Strings or format() method.
F-Strings Formatting
F-Strings are string formatting techniques used in Python to provide us a convenient way of combining numbers, and other expressions inside string literals. The f-strings feature are new in Python and only be found in Python version 3.6.
F-Strings make our code more readable and ease to understand.
We use F-strings by placing an “f” or “F” character in front of a string literal.
Example 19: Combining string, and numbers such as int, float, and boolean (bool) using the f-string formatting technique.
var_str_1 = f"Python is {65} easy to learn {67.9} and easy to understand {True}. empty{{}}"
var_int = 90
var_float = 89.67
var_bool = True
var_str_2 = f"Python is {var_int} easy to learn {var_float} and easy to understand {var_bool} ! {var_bool+var_int}"
print(var_str_1)
print(var_str_2)
Output:
Python is 65 easy to learn 67.9 and easy to understand True. empty{}
Python is 90 easy to learn 89.67 and easy to understand True ! 91
String Formatting using format() Method
String formatting is one of the correct ways of combining string and numbers such as int, float, boolean and etc. In this case, we can use format()
method.
To use the format() method correctly you have to insert placeholders inside the string. The placeholders are specified using the using curly brackets {}
.
Example 20: Combining string, and numbers such as int, float, and boolean (bool) using format()
method.
var_str = "Python is {} easy to learn {} and easy to understand {} "
var_int = 90
var_float = 89.67
var_bool = True
print(var_str.format(var_int,var_float,var_bool))
Output:
Python is 90 easy to learn 89.67 and easy to understand True
The above example uses an empty placeholder while the following examples use named and numerical placeholders when using format()
method.
Example 21: Using format()
method with named placeholders.
var_str = "Python is {int_num} easy to learn {float_num} and easy to understand {bool_num} "
var_int = 90
var_float = 89.67
var_bool = True
print(var_str.format(int_num = var_int, float_num = var_float, bool_num = var_bool))
Output:
Python is 90 easy to learn 89.67 and easy to understand True
Example 22: Using format()
method with numerical placeholders.
var_str = "Python is {1} easy to learn {2} and easy to understand {3} ! {0}"
var_int = 90
var_float = 89.67
var_bool = True
print(var_str.format(var_int, var_float, var_bool,"Amazing"))
Output:
Python is 89.67 easy to learn True and easy to understand Amazing ! 90
Conclusion
This Python tutorial article is dedicated to providing several ways to practice Python Strings including assigning string values to a variable, checking its lengths and type, string slicing, string formatting, and many more. The aim of this tutorial is to provide simplified String Python examples. Further practices of string such as string basics and string methods can also be practiced.