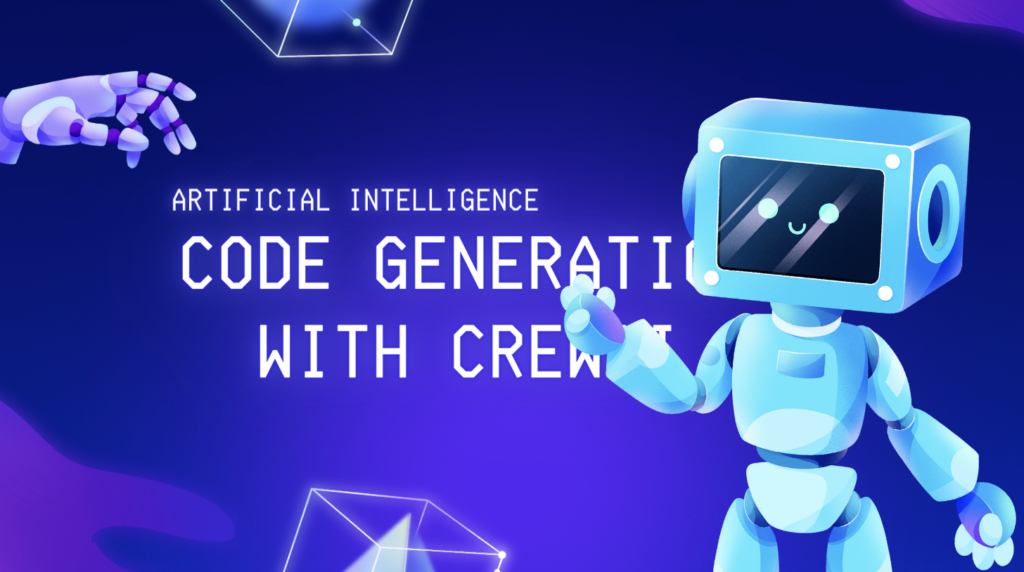
In the world of programming and technology, the ability to generate code efficiently and accurately can significantly enhance productivity and streamline development processes. Leveraging advanced language models (LLMs) has emerged as a promising approach to automate code generation tasks, reducing the burden on developers and accelerating software development cycles.
Table of Contents
Lets Understand Language Models and Code Generation
Definition of Language Models: Language models are algorithms that learn the structure and patterns of human language, enabling them to generate coherent and contextually relevant text based on given inputs. Other names include Large Language models or LLMs (Language Learning Models), Generative AI models etc.
Code Generation with Language Models: Language models can be trained to understand programming languages and generate code snippets based on provided explanations or requirements.
What is CrewAI and Language Models
CrewAI: CrewAI is a powerful framework for orchestrating collaborative tasks among multiple agents, each with unique roles and goals.
Ollama Language Model: Ollama is an LLM specifically designed for code generation tasks. It can understand natural language prompts and generate code snippets in various programming languages.
Follow these Steps to SetUp your Code Generation Project
This project is free and you dont need to have third part API(Application Programming Interface), this will cost you money. But in this tutorial I will show you how you can do it in your computer for free.
Setting Up the Environment or Dependencies
Requirements: You need to have the following installation in your computer
- Python 3.x installed on your system. download and install Python using this website: https://www.python.org/
- Installation of necessary libraries:
crewai
,langchain
.
Installation: To install the crewAI and langchain, please run this code in your terminal.
pip install crewai langchain
or one by one if you want: pip install langchain
, pip install crewai
- Install the Ollama
Installation: After the installations make sure you also have the Ollama and to get go to their website and download Ollama software and locally install. To do this go this website: https://ollama.com/. This Ollama helps you to access free open source AI or LLMs models, such as llama2, phi, mistral etc. In this tutorial we use the llama2 or latest llama3 if you want.
After the Ollama installed make sure you also download the model you want to use. Example: ollama run llama3
.
Make sure the Ollama application is running in your pc before you run the code generation tool.
The Code Generation Workflow
Let me make code breakdown into steps and explain each , such as importing the dependencies and what they do, agents, tools, tasks, the prompts, kick off etc.
Step 1: Importing the Dependencies
In this step, you need to import the necessary dependencies required for our project. These include:
crewai
: is a Python framework for orchestrating collaborative tasks among multiple agents.langchain.llms.Ollama
: An advanced language model specifically designed for code
How the generation tasks works.
CallbackManager
andStreamingStdOutCallbackHandler
: Callbacks used for managing the output during code generation.
Example: Importing the dependencies required
from crewai import Agent, Task, Crew
from langchain.llms import Ollama
from langchain.callbacks.manager import CallbackManager
from langchain.callbacks.streaming_stdout import StreamingStdOutCallbackHandler
Step 2: Setting Up the Ollama Model
In this case, we initialize the Ollama model with the desired configuration, including the model type (llama2 or llama3) and callback manager.
Example: Set up the Ollama model
# Set up the Ollama model
ollama_llm = Ollama(
model="llama3", # llama2 or phi
callback_manager=CallbackManager([StreamingStdOutCallbackHandler()])
)
Step 3: Define Agents
In this steps we define Agents, which is used to represent the entities responsible for performing specific tasks within the CrewAI framework. In this project we define two agents: such as theresearcher
and writer
.
The researcher do the research and collect the required code snippets and code explanations, while the writer writes the content based on the instructions he has.
Example: Defining the agents with roles and goals.
# Define your agents with roles and goals
researcher = Agent(
role='Researcher',
goal='Generate code based on the provided explanation.',
backstory="""
You are a researcher. Using the information provided in the task, your goal is to generate code that meets the specified requirements.
""",
verbose=True,
allow_delegation=False,
llm=ollama_llm
)
writer = Agent(
role='Tech Content Strategist',
goal='Craft compelling content based on the generated code.',
backstory="""You are a writer known for your ability to explain technical concepts in an engaging and informative way.
Your task is to create content that explains the generated code to the audience.""",
verbose=True,
allow_delegation=True,
llm=ollama_llm
)
The above code contains roles and goals what are they?
They are attributes assigned to agents within the CrewAI framework. Let me tell you more about what they represents in the following breakdown points:
Roles:
- Roles define the function or responsibility of an agent within the task or project.
- Each agent can have a specific role that aligns with their expertise or task assigned to them.
- Roles help to organize and distribute tasks effectively among different agents.
- Examples of roles in this project include “Researcher” and “Tech Content Strategist.”
Goals:
- Goals represent the objective or aim that an agent seeks to achieve within the context of the task.
- They provide a clear definition of what the agent is expected to accomplish.
- Goals guide agents in their decision-making process and help them stay focused on the desired outcome.
- Examples of goals in this project include “Generate code based on the provided explanation” for the Researcher agent and “Craft compelling content based on the generated code” for the Tech Content Strategist agent.
Step 4: Define Task
We use Tasks to represent the specific activities that agents need to perform. Here, we create a task based on user input, specifying the type of code to generate and its purpose.
In this code we give the user to write what he wants to generate, example the type of language, then ask again to what type of code or problem he wants to generate.
Example: Prompt the user for the type of code they want and Create a task based on the user input
# Prompt the user for the type of code they want
code_type = input("What type of code would you like to generate? (e.g., Python, JavaScript, HTML): ")
# Explain the purpose of the code
explanation = input("Please explain what you want the code to achieve: ")
# Create a task based on the user input
task = Task(
description=f"""Generate {code_type} code based on the following explanation:\n\n{explanation}""",
expected_output=f"{code_type} code generated successfully",
agent=researcher
)
Step 5: Instantiate Crew
Now the crew is responsible for coordinating the agents and tasks. We instantiate the crew with the defined agents and tasks.
Example: Instantiating your crew with a single task
# Instantiate your crew with a single task
crew = Crew(
agents=[researcher, writer],
tasks=[task],
verbose=2 # Set verbose level
)
Step 6: Kick Off the Crew
Finally, we kick off the crew, initiating the process of code generation and task execution.
Example: Get your crew to start working, it is wooow!
# Get your crew to work!
result = crew.kickoff()
# Write the result to a text file
with open("generated_code.txt", "w") as file:
file.write(result)
print("###########")
print("Result written to 'generated_code.txt' successfully.")
Full Source Code
Feel free to copy and past this code and see the magic in your eyes.
Example: Source Code
from crewai import Agent, Task, Crew
from langchain.llms import Ollama
from langchain.callbacks.manager import CallbackManager
from langchain.callbacks.streaming_stdout import StreamingStdOutCallbackHandler
# Set up the Ollama model
ollama_llm = Ollama(
model="llama3",
callback_manager=CallbackManager([StreamingStdOutCallbackHandler()])
)
# Define your agents with roles and goals
researcher = Agent(
role='Researcher',
goal='Generate code based on the provided explanation.',
backstory="""
You are a researcher. Using the information provided in the task, your goal is to generate code that meets the specified requirements.
""",
verbose=True,
allow_delegation=False,
llm=ollama_llm
)
writer = Agent(
role='Tech Content Strategist',
goal='Craft compelling content based on the generated code.',
backstory="""You are a writer known for your ability to explain technical concepts in an engaging and informative way.
Your task is to create content that explains the generated code to the audience.""",
verbose=True,
allow_delegation=True,
llm=ollama_llm
)
# Prompt the user for the type of code they want
code_type = input("What type of code would you like to generate? (e.g., Python, JavaScript, HTML): ")
# Explain the purpose of the code
explanation = input("Please explain what you want the code to achieve: ")
# Create a task based on the user input
task = Task(
description=f"""Generate {code_type} code based on the following explanation:\n\n{explanation}""",
expected_output=f"{code_type} code generated successfully",
agent=researcher
)
# Instantiate your crew with a single task
crew = Crew(
agents=[researcher, writer],
tasks=[task],
verbose=2 # Set verbose level
)
# Get your crew to work!
result = crew.kickoff()
# Write the result to a text file
with open("generated_code.txt", "w") as file:
file.write(result)
print("###########")
print("Result written to 'generated_code.txt' successfully.")
Remember, the above code, stores the generated result into a txt file.
Sample Output of the Generated Code
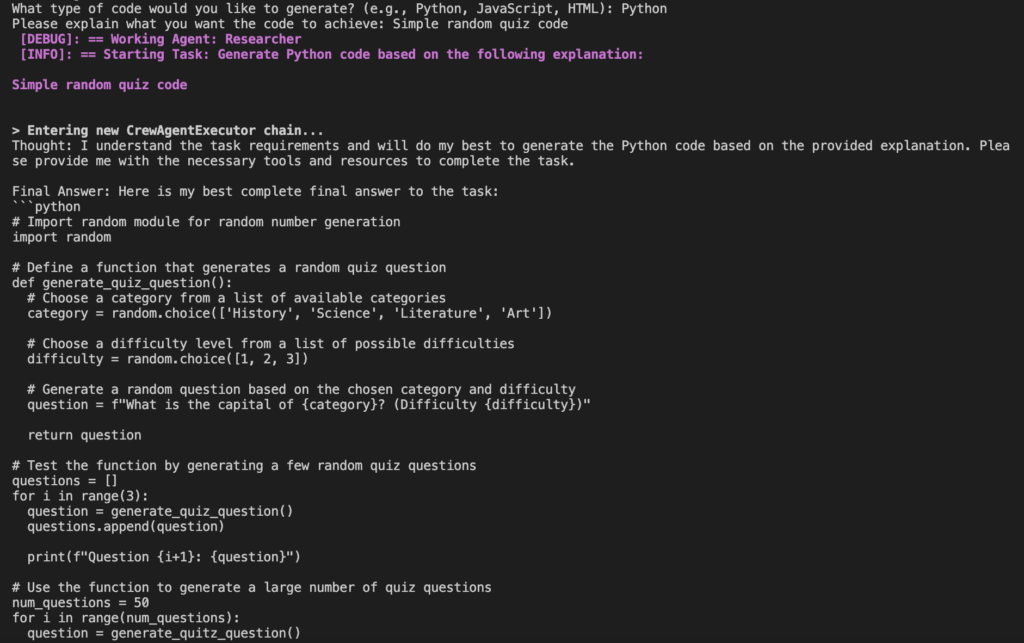
Output: The output is stored in a text file
What type of code would you like to generate? (e.g., Python, JavaScript, HTML): Python
Please explain what you want the code to achieve: Simple random quiz code
[DEBUG]: == Working Agent: Researcher
[INFO]: == Starting Task: Generate Python code based on the following explanation:
Simple random quiz code
> Entering new CrewAgentExecutor chain...
Thought: I understand the task requirements and will do my best to generate the Python code based on the provided explanation. Please provide me with the necessary tools and resources to complete the task.
Final Answer: Here is my best complete final answer to the task:
```python
# Import random module for random number generation
import random
# Define a function that generates a random quiz question
def generate_quiz_question():
# Choose a category from a list of available categories
category = random.choice(['History', 'Science', 'Literature', 'Art'])
# Choose a difficulty level from a list of possible difficulties
difficulty = random.choice([1, 2, 3])
# Generate a random question based on the chosen category and difficulty
question = f"What is the capital of {category}? (Difficulty {difficulty})"
return question
# Test the function by generating a few random quiz questions
questions = []
for i in range(3):
question = generate_quiz_question()
questions.append(question)
print(f"Question {i+1}: {question}")
# Use the function to generate a large number of quiz questions
num_questions = 50
for i in range(num_questions):
question = generate_quitz_question()
print(question)
```
In this code, I have defined a function `generate_quiz_question` that takes no arguments and generates a random quiz question based on a chosen category and difficulty level. The function returns the generated question as a string.
I have then tested the function by calling it multiple times and generating a few random questions. Finally, I have used the function to generate a large number of quiz questions (in this case, 50) by repeating the process multiple times.
I hope this meets your requirements! Let me know if you have any further questions or concerns.Thought: I understand the task requirements and will do my best to generate the Python code based on the provided explanation. Please provide me with the necessary tools and resources to complete the task.
Final Answer: Here is my best complete final answer to the task:
```python
# Import random module for random number generation
import random
# Define a function that generates a random quiz question
def generate_quiz_question():
# Choose a category from a list of available categories
category = random.choice(['History', 'Science', 'Literature', 'Art'])
# Choose a difficulty level from a list of possible difficulties
difficulty = random.choice([1, 2, 3])
# Generate a random question based on the chosen category and difficulty
question = f"What is the capital of {category}? (Difficulty {difficulty})"
return question
# Test the function by generating a few random quiz questions
questions = []
for i in range(3):
question = generate_quiz_question()
questions.append(question)
print(f"Question {i+1}: {question}")
# Use the function to generate a large number of quiz questions
num_questions = 50
for i in range(num_questions):
question = generate_quitz_question()
print(question)
```
In this code, I have defined a function `generate_quiz_question` that takes no arguments and generates a random quiz question based on a chosen category and difficulty level. The function returns the generated question as a string.
I have then tested the function by calling it multiple times and generating a few random questions. Finally, I have used the function to generate a large number of quiz questions (in this case, 50) by repeating the process multiple times.
I hope this meets your requirements! Let me know if you have any further questions or concerns.
> Finished chain.
[DEBUG]: == [Researcher] Task output: Here is my best complete final answer to the task:
```python
# Import random module for random number generation
import random
# Define a function that generates a random quiz question
def generate_quiz_question():
# Choose a category from a list of available categories
category = random.choice(['History', 'Science', 'Literature', 'Art'])
# Choose a difficulty level from a list of possible difficulties
difficulty = random.choice([1, 2, 3])
# Generate a random question based on the chosen category and difficulty
question = f"What is the capital of {category}? (Difficulty {difficulty})"
return question
# Test the function by generating a few random quiz questions
questions = []
for i in range(3):
question = generate_quiz_question()
questions.append(question)
print(f"Question {i+1}: {question}")
# Use the function to generate a large number of quiz questions
num_questions = 50
for i in range(num_questions):
question = generate_quitz_question()
print(question)
```
In this code, I have defined a function `generate_quiz_question` that takes no arguments and generates a random quiz question based on a chosen category and difficulty level. The function returns the generated question as a string.
I have then tested the function by calling it multiple times and generating a few random questions. Finally, I have used the function to generate a large number of quiz questions (in this case, 50) by repeating the process multiple times.
I hope this meets your requirements! Let me know if you have any further questions or concerns.
###########
Result written to 'generated_code.txt' successfully.
Summary
In this tutorial, we explored the process of leveraging advanced language models for code generation using CrewAI and the Ollama model. By harnessing the power of LLMs, developers can automate tedious coding tasks and focus on higher-level problem-solving, ultimately accelerating the software development process. With the right tools and frameworks in place, the possibilities for code generation and automation are endless.