
Are you intrigued by the intersection of finance and technology? Imagine being able to fetch detailed financial information about top companies and enhance this data using advanced language models (LLM), all with just a few steps and few lines of Python code.
In this important article, we will dive into the fascinating world of financial data analysis by leveraging the power of yfinance
for data retrieval and Langchain’s Ollama
for sophisticated data interpretation.
Whether you’re a financial analyst, a tech enthusiast, or just someone looking to explore the potential of AI in finance, this guide will walk you through every step to create a powerful tool for financial insights. Let’s get started!
Table of Contents
Libraries You Need to Install
yfinance
: A popular library for accessing financial data from Yahoo Finance.langchain
: A library that provides tools to work with language models.ollama
: Part of the Langchain library, it helps to interface with specific LLMs.
Installing the Libraries
You can install these libraries using pip: pip install yfinance langchain ollama
Make sure to download the ollama in your machine and also download the specific model you want, here we use llama3. In short follow the below steps:
- Downloading Ollama: To get or download this module, go to the Ollama website and download the software.
- Installing Ollama: Read the instructions written in their page on how to install the ollama.
- Downloading specific model (LLaMA3 Model): Go to your terminal or CMD and run this command:
ollama run llama3
- NOW YOU HAVE OLLAMA AND LLAMA3 model. congratulations.
What These Libraries Do
yfinance
: This library is used to fetch financial data such as stock prices, company information, historical data, etc.langchain
: This library facilitates the use of language models for various natural language processing tasks.ollama
: A component oflangchain
that interfaces with specific language models to enhance and analyze data.
Step-by-Step Explanation and Code Segments
Step 1: Import Libraries
import yfinance as yf
from langchain.llms import Ollama
from langchain.callbacks.manager import CallbackManager
from langchain.callbacks.streaming_stdout import StreamingStdOutCallbackHandler
We import the necessary libraries for fetching stock data and interfacing with the language model.
Step 2: Define the Function to Get Company Information
def get_company_info(ticker):
stock = yf.Ticker(ticker)
info = stock.info
company_info = {
"Name": info.get('shortName', 'N/A'),
"Sector": info.get('sector', 'N/A'),
"Industry": info.get('industry', 'N/A'),
"Country": info.get('country', 'N/A'),
"Market Cap": info.get('marketCap', 'N/A'),
"Dividend Yield": info.get('dividendYield', 'N/A'),
"52-Week High": info.get('fiftyTwoWeekHigh', 'N/A'),
"52-Week Low": info.get('fiftyTwoWeekLow', 'N/A'),
"P/E Ratio": info.get('trailingPE', 'N/A'),
"EPS": info.get('trailingEps', 'N/A'),
"Earnings Date": info.get('earningsDate', 'N/A'),
"CEO": info.get('ceo', 'N/A'),
"Website": info.get('website', 'N/A'),
"Description": info.get('longBusinessSummary', 'N/A'),
"Employees": info.get('fullTimeEmployees', 'N/A'),
"Headquarters": info.get('city', 'N/A') + ", " + info.get('state', 'N/A')
}
return company_info
This function fetches detailed information about a company using its ticker symbol. The yf.Ticker
object retrieves various attributes that are stored in a dictionary.
Step 3: Main Function to Fetch and Display Information
def main():
tickers = ['AAPL'] # List of company tickers to fetch information for
all_company_info = []
for ticker in tickers:
info = get_company_info(ticker)
all_company_info.append(info)
return all_company_info
if __name__ == "__main__":
all_info = main()
# Convert the list of company information to a single string for LLM input
info_str = "\n".join([str(info) for info in all_info])
# Set up the Ollama model
ollama_llm = Ollama(
model="llama3",
callback_manager=CallbackManager([StreamingStdOutCallbackHandler()])
)
# Make the request to the LLM with the gathered information
response = ollama_llm(f""" Present the provided information in a meaningful way and guess and fill the missing data: {info_str}""")
print("###########")
print(response)
The main
function initializes a list of company tickers and uses the get_company_info
function to fetch their information. This information is then formatted as a string and passed to the Ollama
LLM for further processing.
Step 4: Processing with the LLM
The Ollama
model is set up with a callback manager to handle responses. The information collected is passed to the LLM to enhance and fill in any missing details. The response is then printed out.
Full Script
Here is the complete script:
import yfinance as yf
from langchain.llms import Ollama
from langchain.callbacks.manager import CallbackManager
from langchain.callbacks.streaming_stdout import StreamingStdOutCallbackHandler
def get_company_info(ticker):
stock = yf.Ticker(ticker)
info = stock.info
company_info = {
"Name": info.get('shortName', 'N/A'),
"Sector": info.get('sector', 'N/A'),
"Industry": info.get('industry', 'N/A'),
"Country": info.get('country', 'N/A'),
"Market Cap": info.get('marketCap', 'N/A'),
"Dividend Yield": info.get('dividendYield', 'N/A'),
"52-Week High": info.get('fiftyTwoWeekHigh', 'N/A'),
"52-Week Low": info.get('fiftyTwoWeekLow', 'N/A'),
"P/E Ratio": info.get('trailingPE', 'N/A'),
"EPS": info.get('trailingEps', 'N/A'),
"Earnings Date": info.get('earningsDate', 'N/A'),
"CEO": info.get('ceo', 'N/A'),
"Website": info.get('website', 'N/A'),
"Description": info.get('longBusinessSummary', 'N/A'),
"Employees": info.get('fullTimeEmployees', 'N/A'),
"Headquarters": info.get('city', 'N/A') + ", " + info.get('state', 'N/A')
}
return company_info
def main():
tickers = ['AAPL'] # List of company tickers to fetch information for
all_company_info = []
for ticker in tickers:
info = get_company_info(ticker)
all_company_info.append(info)
return all_company_info
if __name__ == "__main__":
all_info = main()
# Convert the list of company information to a single string for LLM input
info_str = "\n".join([str(info) for info in all_info])
# Set up the Ollama model
ollama_llm = Ollama(
model="llama3",
callback_manager=CallbackManager([StreamingStdOutCallbackHandler()])
)
# Make the request to the LLM with the gathered information
response = ollama_llm(f""" Present the provided information in a meaningful way and guess and fill the missing data: {info_str}""")
print("###########")
print(response)
Sample Output
Feel the magic and see the result. Remember AI or LLMS is in your hand and you don’t need to rely single tutorial, make your app do the magic and find ways to alter the code.
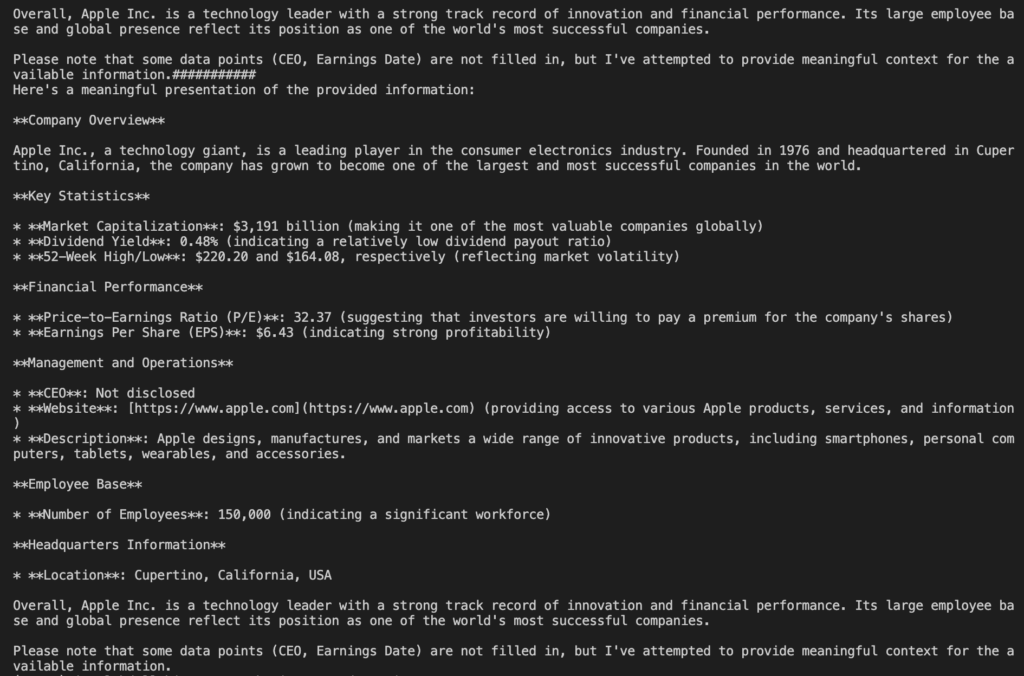
DO MORE THAN THAT
How to Do Customization and Hints to Take
1. Adding More Companies
You can expand the tickers
list to include more companies, such as:
tickers = ['AAPL', 'MSFT', 'GOOGL', 'AMZN', 'TSLA', 'NVDA']
2. Error Handling
Enhance the get_company_info
function with error handling to manage situations where data might be unavailable:
def get_company_info(ticker):
try:
stock = yf.Ticker(ticker)
info = stock.info
company_info = {
"Name": info.get('shortName', 'N/A'),
"Sector": info.get('sector', 'N/A'),
"Industry": info.get('industry', 'N/A'),
"Country": info.get('country', 'N/A'),
"Market Cap": info.get('marketCap', 'N/A'),
"Dividend Yield": info.get('dividendYield', 'N/A'),
"52-Week High": info.get('fiftyTwoWeekHigh', 'N/A'),
"52-Week Low": info.get('fiftyTwoWeekLow', 'N/A'),
"P/E Ratio": info.get('trailingPE', 'N/A'),
"EPS": info.get('trailingEps', 'N/A'),
"Earnings Date": info.get('earningsDate', 'N/A'),
"CEO": info.get('ceo', 'N/A'),
"Website": info.get('website', 'N/A'),
"Description": info.get('longBusinessSummary', 'N/A'),
"Employees": info.get('fullTimeEmployees', 'N/A'),
"Headquarters": info.get('city', 'N/A') + ", " + info.get('state', 'N/A')
}
return company_info
except Exception as e:
return {"Error": str(e)}
3. Using Other Data Points
You can add more fields from the yfinance
API to the company_info
dictionary, such as revenue, profit margins, or beta:
company_info = {
...
"Revenue": info.get('totalRevenue', 'N/A'),
"Profit Margin": info.get('profitMargins', 'N/A'),
"Beta": info.get('beta', 'N/A')
}
4. Improving the LLM Request
The request made to the LLM can be improved by providing a more detailed prompt. For example:
response = ollama_llm(f"""Using the provided company information, generate a comprehensive report highlighting the key financial metrics and business overview for each company. Also, suggest possible trends or insights based on the data: {info_str}""")
import yfinance as yf
from langchain.llms import Ollama
from langchain.callbacks.manager import CallbackManager
from langchain.callbacks.streaming_stdout import StreamingStdOutCallbackHandler
def get_company_info(ticker):
try:
stock = yf.Ticker(ticker)
info = stock.info
company_info = {
"Name": info.get('shortName', 'N/A'),
"Sector": info.get('sector', 'N/A'),
"Industry": info.get('industry', 'N/A'),
"Country": info.get('country', 'N/A'),
"Market Cap": info.get('marketCap', 'N/A'),
"Dividend Yield": info.get('dividendYield', 'N/A'),
"52-Week High": info.get('fiftyTwoWeekHigh', 'N/A'),
"52-Week Low": info.get('fiftyTwoWeekLow', 'N/A'),
"P/E Ratio": info.get('trailingPE', 'N/A'),
"EPS": info.get('trailingEps', 'N/A'),
"Earnings Date": info.get('earningsDate', 'N/A'),
"CEO": info.get('ceo', 'N/A'),
"Website": info.get('website', 'N/A'),
"Description": info.get('longBusinessSummary', 'N/A'),
"Employees": info.get('fullTimeEmployees', 'N/A'),
"Headquarters": info.get('city', 'N/A') + ", " + info.get('state', 'N/A'),
"Revenue": info.get('totalRevenue', 'N/A'),
"Profit Margin": info.get('profitMargins', 'N/A'),
"Beta": info.get('beta', 'N/A')
}
return company_info
except Exception as e:
return {"Error": str(e)}
def main():
tickers = ['AAPL', 'MSFT', 'GOOGL', 'AMZN', 'TSLA', 'NVDA'] # List of company tickers to fetch information for
all_company_info = []
for ticker in tickers:
info = get_company_info(ticker)
all_company_info.append(info)
return all_company_info
if __name__ == "__main__":
all_info = main()
# Convert the list of company information to a single string for LLM input
info_str = "\n".join([str(info) for info in all_info])
# Set up the Ollama model
ollama_llm = Ollama(
model="llama3",
callback_manager=CallbackManager([StreamingStdOutCallbackHandler()])
)
# Make the request to the LLM with the gathered information
response = ollama_llm(f"""Using the provided company information, generate a comprehensive report highlighting the key financial metrics and business overview for each company. Also, suggest possible trends or insights based on the data: {info_str}""")
print("###########")
print(response)
Summary
In this article, I demonstrated how to fetch and enhance company information using Python and a large language model. By combining yfinance
for data retrieval and Langchain’s Ollama
for data enhancement, we can create a powerful tool for financial analysis and reporting.
https://learnpythoneasily.com/dynamic-web-pages-with-crewai/