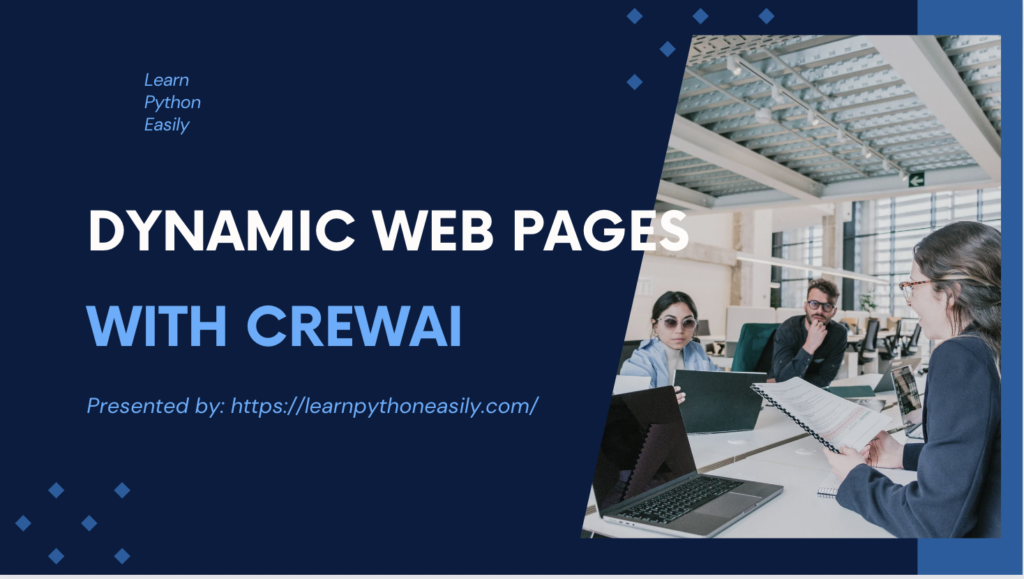
In the digital age, having a captivating online presence is essential for individuals and businesses alike. Whether it’s a professional CV, an engaging portfolio, or an interactive blog, creating a compelling web page requires a combination of HTML structure, CSS styling, and JavaScript functionality. However, not everyone possesses the coding skills or the time to develop these elements from scratch. That’s where advanced language models (LLMs) and collaboration tools like CrewAI come into play.
Table of Contents
What We do in this Tutorial
In this blog post, we’ll explore how to leverage CrewAI, an AI-powered collaboration platform, along with advanced language models like Ollama, to generate dynamic web pages effortlessly. We’ll walk through the process step by step, from setting up the project to executing tasks and generating the final output.
Task Break Down
Let’s break down the code and guide you on how generating the required tasks can be done:
Let’s break down each library and what it contributes to the code:
- crewai: This library provides functionalities for creating and managing teams of virtual agents (or “crew”) to perform tasks collaboratively. It allows for the coordination of multiple agents with specific roles and responsibilities.
- langchain: This library offers tools and utilities for natural language processing (NLP) tasks, particularly in the context of language model training and inference. It includes components for working with large language models (LLMs), such as Ollama.
- Ollama (part of langchain): Ollama is a large language model (LLM) provided by Langchain. It serves as the backbone for generating text-based content, such as code snippets or written explanations, based on given inputs or prompts. In this code, Ollama is used for generating HTML, CSS, and JavaScript code based on user requirements.
Step 1: Install Them
To install these libraries, you can use Python’s package manager pip. Follwo this instruction to install them:
pip install crewai
pip install langchain
or pip install -U langchain-community
Step 2: Setting up the Environment:
The first step is to set up the environment by importing the necessary libraries and initializing the Ollama model.
Setup libraries: Import necessary libraries and set up the Ollama model and agents.
Example: Importing the libraries
from crewai import Agent, Task, Crew
from langchain.llms import Ollama
from langchain.callbacks.manager import CallbackManager
from langchain.callbacks.streaming_stdout import StreamingStdOutCallbackHandler
Initializing the Ollama model: Ollama acts as our language model, providing natural language understanding and code generation capabilities.
Example: Ollama Setup
# Set up the Ollama model
ollama_llm = Ollama(
model="llama3",
callback_manager=CallbackManager([StreamingStdOutCallbackHandler()])
)
Step 3: Define the Agents with Roles and Goals
Agent Definition: Make sure to define agents for HTML development, CSS styling, and JavaScript development.
Each agent has a specific goal and backstory, guiding their behavior during task execution.
In this code we will be having multiple agents (three agents): one for generating HTML, one for CSS, and one for JavaScript.
Each agent’s goal is to generate their respective part of the web page code based on the provided explanation.
Example: Define agents with roles and goals
# Define agents with roles and goals
html_agent = Agent(
role='HTML Developer',
goal='Generate HTML code for the web page.',
backstory="""You are an HTML developer. Your goal is to create the structure and content of the web page using HTML.""",
verbose=True,
allow_delegation=False,
llm=ollama_llm
)
css_agent = Agent(
role='CSS Stylist',
goal='Create CSS code for styling the web page.',
backstory="""You are a CSS stylist. Your task is to design the appearance and layout of the web page using CSS.""",
verbose=True,
allow_delegation=False,
llm=ollama_llm
)
js_agent = Agent(
role='JavaScript Developer',
goal='Develop JavaScript code for interactive features.',
backstory="""You are a JavaScript developer. Your objective is to implement interactive elements and functionality on the web page.""",
verbose=True,
allow_delegation=False,
llm=ollama_llm
)
Step 4: Prompt the User for Web Page Details
User Input: Ask the user for the type of web page they want to create and provide a brief description of the content and features.
We’ll ask or prompt the user to specify the type of web page they want (e.g., CV, portfolio, blog). Based on the user’s choice, we’ll generate content accordingly.
Example: Prompt the user for the type of web page they want
# Prompt the user for the type of web page they want
web_page_type = input("What type of web page would you like to create? (e.g., CV, portfolio, blog): ")
# Provide an explanation for the web page content
web_page_content = input("Please provide a brief description of the content and features you want on the web page: ")
Step 5: Task Creation: Generate HTML, CSS, and JavaScript:
Create tasks for each agent based on the user input, specifying the descriptions and expected outputs.
Each agent will generate the code for their respective part (HTML, CSS, JavaScript) based on the user’s input and their roles. The content will be synthesized using the Ollama model (llama3).
Example: Create tasks for each agent based on the user input
# Create tasks for each agent based on the user input
html_task = Task(
description=f"""Generate HTML code for a {web_page_type} web page based on the following content:\n\n{web_page_content}""",
expected_output="HTML code generated successfully",
agent=html_agent
)
css_task = Task(
description="Create CSS code to style the web page.",
expected_output="CSS code generated successfully",
agent=css_agent
)
js_task = Task(
description="Develop JavaScript code for interactive features.",
expected_output="JavaScript code generated successfully",
agent=js_agent
)
Step 6: Crew Instantiation
Instantiate the crew with the defined agents and tasks.
Example: Instantiate the crew with the tasks
# Instantiate the crew with the tasks
crew = Crew(
agents=[html_agent, css_agent, js_agent],
tasks=[html_task, css_task, js_task],
verbose=2
)
Step 7: Task Execution
Execute the tasks using the kickoff()
function.
Example: Execute the tasks
# Execute the tasks
result = crew.kickoff()
Step 8: Combine and Save the Generated Code
We finally combine the generated HTML, CSS, and JavaScript code into a single web page.
After all agents have completed their tasks, this code combines the generated HTML, CSS, and JavaScript into a single web page.
Example: Combine the generated code into a single web page. We use image because, the page ignores the html code, please type this code if you want.
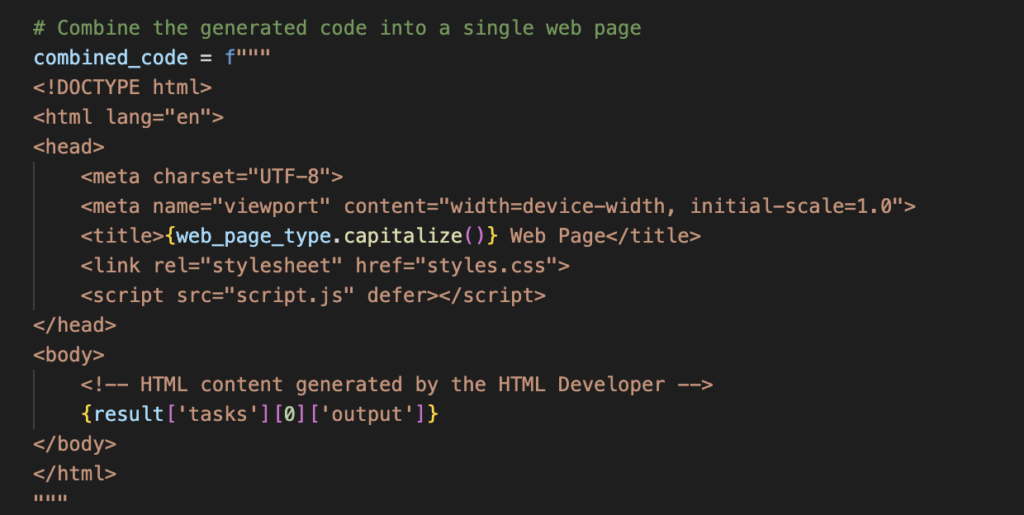
Step 9: Saving Output
Finally, we’ll save the combined code as an HTML file. This combines the generated code as an HTML file.
Example: Save the combined code as an HTML file
# Save the combined code as an HTML file
with open(f"{web_page_type.lower()}_page.html", "w") as file:
file.write(combined_code)
Step 10: Print Output
Print a success message indicating the completion of the process.
Example: Printing the Output
print("###########")
print(f"{web_page_type.capitalize()} web page generated successfully. Check '{web_page_type.lower()}_page.html' for the output.")
Get The Full Source code HERE!
Example: Full Source code
from crewai import Agent, Task, Crew
from langchain.llms import Ollama
from langchain.callbacks.manager import CallbackManager
from langchain.callbacks.streaming_stdout import StreamingStdOutCallbackHandler
# Set up the Ollama model
ollama_llm = Ollama(
model="llama3",
callback_manager=CallbackManager([StreamingStdOutCallbackHandler()])
)
# Define agents with roles and goals
html_agent = Agent(
role='HTML Developer',
goal='Generate HTML code for the web page.',
backstory="""You are an HTML developer. Your goal is to create the structure and content of the web page using HTML.""",
verbose=True,
allow_delegation=False,
llm=ollama_llm
)
css_agent = Agent(
role='CSS Stylist',
goal='Create CSS code for styling the web page.',
backstory="""You are a CSS stylist. Your task is to design the appearance and layout of the web page using CSS.""",
verbose=True,
allow_delegation=False,
llm=ollama_llm
)
js_agent = Agent(
role='JavaScript Developer',
goal='Develop JavaScript code for interactive features.',
backstory="""You are a JavaScript developer. Your objective is to implement interactive elements and functionality on the web page.""",
verbose=True,
allow_delegation=False,
llm=ollama_llm
)
# Prompt the user for the type of web page they want
web_page_type = input("What type of web page would you like to create? (e.g., CV, portfolio, blog): ")
# Provide an explanation for the web page content
web_page_content = input("Please provide a brief description of the content and features you want on the web page: ")
# Create tasks for each agent based on the user input
html_task = Task(
description=f"""Generate HTML code for a {web_page_type} web page based on the following content:\n\n{web_page_content}""",
expected_output="HTML code generated successfully",
agent=html_agent
)
css_task = Task(
description="Create CSS code to style the web page.",
expected_output="CSS code generated successfully",
agent=css_agent
)
js_task = Task(
description="Develop JavaScript code for interactive features.",
expected_output="JavaScript code generated successfully",
agent=js_agent
)
# Instantiate the crew with the tasks
crew = Crew(
agents=[html_agent, css_agent, js_agent],
tasks=[html_task, css_task, js_task],
verbose=2
)
# Execute the tasks
result = crew.kickoff()
# Combine the generated code into a single web page
combined_code = f"""
{web_page_type.capitalize()} Web Page
{result['tasks'][0]['output']}
"""
# Save the combined code as an HTML file
with open(f"{web_page_type.lower()}_page.html", "w") as file:
file.write(combined_code)
print("###########")
print(f"{web_page_type.capitalize()} web page generated successfully. Check '{web_page_type.lower()}_page.html' for the output.")
Sample Output

Conclusion
With CrewAI and advanced language models, the process of creating dynamic web pages becomes more accessible and efficient. Whether you’re a developer looking to streamline your workflow or a novice seeking to build your online presence, this combination of AI-powered tools can revolutionize the way web development is approached.